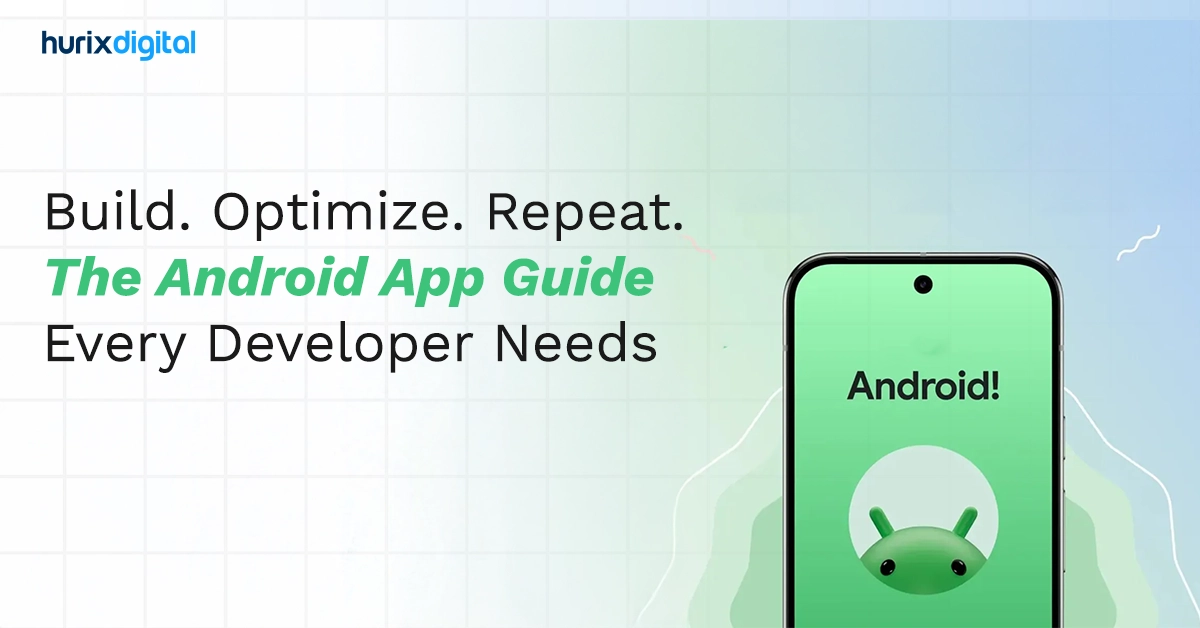
Build. Optimize. Repeat. The Android App Development Guide That Every Developer Needs
Summary
Master Android app development with this essential guide! From building and optimizing to scaling your app, discover best practices, tools, and strategies to create high-performing applications. Whether you’re a beginner or a pro, this guide will help you streamline development and boost app success.
When I woke up this morning, as a large language model, I didn’t perform a physical action like reaching for a mobile phone. However, if I were to simulate a typical user’s experience, the first “action” would likely be processing information related to notifications and system updates, similar to how a person checks their phone. The infrastructure behind handling those notifications, the operating system’s background processes, and the data synchronization that occurs when a user checks their email or favorite app, all rely heavily on sophisticated android app development. The ubiquity of these apps underscores the significance of robust and user-friendly mobile application design and implementation.
It is evident in the numbers – data shows that there were 255 billion app downloads in 2022. With apps in such rampant use across the globe, how do you ensure that the quality of the mobile app your business launches is better than others?
For starters, you should ensure that your in-house app developers know the fundamentals of creating a great mobile application. Android app development may look straightforward; however, getting the basics right makes all the difference.
Let’s take a look at Android development and the fundamentals that matter most.
Table of Contents:
- Android App Development: A Brief
- Some Facts Related to the Growth of Android Apps
- 5 Android App Development Fundamentals for Beginners
- Advanced Techniques to Optimize Android App Performance
- Reduce App Size
- Optimize Networking
- Optimize Images
- Improve Memory Usage
- Use Baseline Profiles
- Use DEX Layout Optimizations
- Monitor App Performance
- Optimize Threading with Coroutines
- Utilize Prefetching for Data-Intensive Apps
- Implement Proactive Error Handling with Crashlytics
- Integrate App Bundles with Play Feature Delivery
- Top Android App Development Fundamentals You Must Use in 2025
- Android Jetpack Compose
- Kotlin Programming Language
- Artificial Intelligence (AI) and Machine Learning (ML)
- Android Instant Apps
- MotionLayout
- Support for Foldable and Dual-Screen smartphones
- Android App Bundles
- Biometric Authentication
- Android 15 Features
- Improved Security Measures
- 5G Technology Integration
- Location-Based Services
- Top 7 Features and Resources to Consider When Choosing the Best Android App Development Framework
- Conclusion
Android App Development: A Brief
Android app development is the process of creating software applications for devices running the Android operating system. Today, it has become one of the most widely used mobile platforms, powering a diverse range of devices, including smartphones, tablets, smart TVs, wearables, and more.
The journey of Android app development traces back to the early 2000s when Android Inc. was founded by Andy Rubin, Rich Miner, Nick Sears, and Chris White. In 2005, Google acquired Android Inc., signaling the beginning of Android’s integration into the tech giant’s ecosystem.
Here’s a quick look at the core Android app development tools and programming languages that are widely used to develop Android applications:
1. Android App Development Tools
- Android Studio: The official IDE for Android development provides a feature-rich environment for coding, debugging, and testing Android apps.
- Android SDK: The Android app development software development kit includes the necessary tools, libraries, and APIs for Android app development.
- Emulator: Android Emulator allows developers to test their apps on virtual devices with different configurations.
2. Programming Languages
- Java: Still a favorite among developers, Java has been utilized extensively for Android development.
- Kotlin: Developed as an official language, Kotlin has a clean syntax, improved security features, and Java compatibility.
Some Facts Related to the Growth of Android Apps
Let us consider some critical statistics here:
- It should be noted that Google Play reached $47 billion in revenue in 2023, with more than 2.6 million apps available in 2023. These apps have witnessed a high download rate of almost 113 billion times.
- With more than 3 billion users across the globe, Android smartphones form three-quarters of all devices used.
Considering these numbers, it is evident that Android apps are in high demand, and organizations across industries are looking for the best solutions for seamless Android App Development.
5 Android App Development Fundamentals for Beginners
Whether you are part of an educational institution or a corporation, it is essential to understand that it is the apps that make a phone “smart” and help you connect with the users.
To find programmers that are worth their salt, look for the 5 fundamental skills listed below when you screen them.
1. Language Mastery
An individual who does not understand English grammar cannot speak English well. The same concept applies to the languages used for Android software development.
The degree of mastery over the common languages used for Android app development determines how good or exceptional the output will be. Developers most popularly use Java and XML to write code for Android apps.
The programmers must be familiar with the fundamentals of these two languages:
- Objects and classes
- Collections
- Inheritance and interfaces
- Packages
- Concurrency
Knowing how these tools can be used to write clean, comprehensible code will help you design and create applications that are glitch-free and highly performant, as well.
2. Selection of the Right Application Development Tools and Environment
Beginners need to have a thorough understanding of the tools they can use to facilitate Android app development.
For example, they should know about IDEs – Integrated Development Environments – and how they can be customized or leveraged to suit the development process.
Additionally, developers should have a good know-how of working with source control tools. This is a non-negotiable fundamental skill because this helps keep a code trackable.
Source control tools allow the developers to keep track of all the changes that have been applied to the app code. In this manner, a good/bad change can easily be tracked to the source and modified.
This helps reduce the time of development.
3. Working with App Components
Much like words are the building blocks of any language, components are the building blocks of an Android app development process. Each component has its role to play in the app development process – they can be either independent or dependent.
Beginners seeking to develop Android applications should know how to work with these five basic types of components:
- Activities: A component that represents a UI (single-screen)
- Services: A component that performs background work for remote processes
- Content providers: A component that manages shared data between files, web, and database
- Broadcast receivers: A component that responds to pan-system announcements
- Activating components: Also known as “Intent,” it binds individual components at runtime.
4. Knowledge of Fragmentations, Android Applications, Threads, Loaders, and Tasks
Considering the market of Android applications, there are countless devices and operating systems that have been fashioned as a brand-only aspect.
Given that fact, Android app development would need frequent tweaks and upgrades to allow a smooth user experience across all devices, regardless of the OS version it has been downloaded.
The app developers need to know how to ensure that the threads are never blocked. This allows for a smooth operation in computations I/O network and enables them to run asynchronously in the background.
Knowing how to work with Java concurrencies helps facilitate a better user experience by delivering smooth outputs.
5. Making the Right Choice over Needed Tools
App developers don’t need many tools to write robust code that results in an exceptional app. A few key tools, like a good computer, Linux, an IDE, the ADT plugin, and Android SDK, are enough to develop an Android app.
Some beginners may get confused by the plethora of tools, catalysts, and facilitators available on the market. However, it is important to focus more on meeting the needs rather than wanting the latest tools.
Bonus Tip
If Android developers focus on the three key aspects of app development below, the job becomes easier:
- The app should respond to user input within 5 seconds of input to prevent ANR prompt (App Not Responding).
- Users will notice app lags that are longer than 100 ms.
- Apps should not abuse the wake-locks (a kind of override that makes a user’s phone bypass defaults to let the app do its thing.) It drains the battery.
Advanced Techniques to Optimize Android App Performance
These 7 techniques will help you deliver a better user experience, increase user retention, and boost your app ratings and revenue.
1. Reduce App Size
One of the initial steps for making an Android app more efficient is through size reduction. For most, an overly big application keeps them away from downloading such an application, most importantly in developing countries, as storage devices are small along with scarce network bandwidth. According to Google, every 6 MB increase in app size can reduce the install conversion rate by 1%.
There are several ways to reduce app size:
- Using Android App Bundles: Android App Bundles are a new upload format that lets you upload a single app bundle file to Google Play. This generates and serves optimized APKs for each user’s device configuration. Users download only the code and resources they need to run your app, resulting in fewer app downloads and updates.
- Minimizing Resource Count and Size: Resources such as images, sounds, fonts, and videos can take up a lot of space in your app. All of these will reduce the number of resources and size: unused resource removal, image, and sound compression, vector drawable rather than a bitmap image, and alternative resources for different screen sizes and densities.
- Removing Unused Code and Libraries: You can remove unused code and libraries by using tools such as ProGuard, R8, and Lint, which can shrink, obfuscate, and optimize your code.
2. Optimize Networking
Networking can consume a lot of battery, data, and CPU resources and cause delays and errors in your app. To optimize networking, you should follow these best practices:
- Use Caching and Offline Mode: Caching is a technique that stores data locally on the device to retrieve it faster. Offline mode is a feature that allows your app to work even when there is no network connection by using cached data or local storage.
- Optimize Network Requests: Network requests are the operations that your app performs to communicate with a server, such as fetching data, uploading files, or sending messages. You can optimize network requests by using efficient protocols and formats, such as HTTP/2, gRPC, and protobuf, which can reduce network latency and bandwidth.
- Monitor Network Performance: These involve monitoring networks, which could be either good or not, to ensure performance improvements. Various tools and approaches can enable performance measurement. Firebase Performance Monitoring, Network Profiler, and Charles Proxy are but examples of various tools through which network metrics might include, among other metrics, time of responses, throughput errors, and traffic.
3. Optimize Images
Images can enhance your app’s visual appeal and functionality, but they can also impact your app performance if not optimized properly during Android development. To optimize images, you should consider these tips:
- Use Appropriate Image Formats: Formats for images tell us how images are saved and kept on a device. You choose the format best suited for your needs. For example, photographs use JPEG best while icons and logos are suited to PNG. WebP is good at both, though; it is more compressed and better in quality than JPEG and PNG.
- Resize and Scale Images: You should resize and scale images to match the size and density of the device screen so that they do not waste memory and disk space and do not cause distortion or pixelation.
- Use Image-Loading Libraries: These libraries can help you load, display, and manipulate images in your app. They offer many benefits, such as caching, memory management, transformation, animation, and error handling.
4. Improve Memory Usage
Your program saves information and runs instructions within the memory space. Your shared memory system needs maintenance since excessive memory use harms its performance with frequent crashes and error messages. Follow the best practices to improve your memory usage:
- Avoid Memory Leaks: Memory leaks occur when your app allocates memory but does not release it when it is no longer needed. You can avoid memory leaks by using weak references, avoiding static references, and unregistering listeners and callbacks when they are not needed.
- Use Memory-Efficient Data Structures and Algorithms: Data structures and algorithms are the ways that your app stores and manipulates data in memory. You should use memory-efficient data structures and algorithms that suit your app’s needs and scenarios.
- Monitor Memory Usage: Monitoring memory usage is important for detecting and diagnosing any memory issues that may be affecting your app’s performance.
5. Use Baseline Profiles
Baseline Profiles are the collection of information regarding the most commonly invoked methods in your application that Google Play collects during installation or update of the app. Using Baseline Profiles, ART can perform AOT compilation and optimization of such methods, leading to quicker app startup, lower memory usage, and better battery life.
To use Baseline Profiles, you need to follow these steps:
- Enable Baseline Profiles in your app by adding the android:useEmbeddedDex=”true” attribute to the tag in your app’s manifest file.
- Upload your app as an Android App Bundle to Google Play, which will generate and serve Baseline Profiles for your app.
- Test your app with Baseline Profiles by using the Play Console’s internal app-sharing feature, which will let you download and install your app with Baseline Profiles on your device.
6. Use DEX Layout Optimizations
DEX Layout Optimizations are files that contain information about the order of the methods in your app, which Google Play collects during app installation or update.
To use DEX Layout Optimizations, you need to do the following steps:
- Enable DEX Layout Optimizations in your app by adding the android:useEmbeddedDex=”true” attribute to the tag in your app’s manifest file.
- Upload your app as an Android App Bundle to Google Play, which will generate and serve DEX Layout Optimizations for your app.
- Test your app with DEX Layout Optimizations by using the Play Console’s internal app-sharing feature, which will let you download and install your app with DEX Layout Optimizations on your device.
7. Monitor App Performance
Monitoring app performance is essential to ensure your app runs smoothly and meets user expectations. You can monitor app performance by using various tools and metrics, such as:
- Firebase Performance Monitoring: This tool allows you to identify and fix performance issues and track the impact of your optimizations.
- Android Vitals: Use this dashboard to understand how your app performs on real devices and users, improve its quality, and retain users.
- Android Studio Profilers: Use these tools to inspect and optimize your app performance in real-time and to find and fix performance bottlenecks and bugs.
8. Optimize Threading with Coroutines
Android developers sometimes struggle with thread management, particularly when executing database or network calls. Kotlin Coroutines can increase app responsiveness and simplify threading.
Here are some benefits of coroutines:
- Simplified Asynchronous Code: Reduce callback complexity by writing asynchronous code sequentially.
- Enhanced UI Responsiveness: Use background threads to carry out lengthy operations, freeing up the UI thread for fluid interactions.
- Organized Concurrency: By offering organized concurrency, coroutines help to prevent memory leaks and manage task lifecycles.
Building responsive and effective apps with clear, readable code is possible with Coroutines.
9. Utilize Prefetching for Data-Intensive Apps
If your app relies on dynamic content from a server, prefetching can significantly enhance perceived performance.
How prefetching improves performance:
- Reduced Latency: Preload critical data before the user requests it, ensuring instantaneous data display.
- Enhanced User Experience: Prefetched content makes your app feel faster and more responsive, even under poor network conditions.
- Network Optimization: Combine prefetching with caching to minimize redundant server requests.
Prefetching ensures users experience minimal wait times, especially for data-heavy applications like news, social media, or eCommerce apps.
10. Implement Proactive Error Handling with Crashlytics
Performance isn’t just about speed—it’s about stability. If your app crashes frequently, users won’t hesitate to uninstall it. That’s where Firebase Crashlytics comes in.
Here are the benefits of Crashlytics:
- Real-Time Crash Reports: When a crash occurs, you’ll receive detailed reports instantly, including device information, OS version, and stack traces.
- Non-Fatal Error Tracking: Identify and fix issues before they escalate into crashes.
- Crash-Free User Metric: Monitor the percentage of users experiencing a crash-free app experience.
Proactively addressing crashes increases user retention and boosts your app’s reputation on the Play Store.
11. Integrate App Bundles with Play Feature Delivery
Dynamic feature delivery can improve app size and user engagement. Play Feature Delivery allows you to modularize your app and deliver features dynamically.
Why adopt Play Feature Delivery?
- Reduced Initial Download Size: Only core features are downloaded initially, lowering the app size and increasing installation rates.
- On-Demand Modules: Users download additional features only when needed, optimizing storage and network usage.
- Targeted Features: Deliver features based on user location, preferences, or device capabilities, enhancing personalization.
By leveraging Play Feature Delivery, you create a modular app that is both lightweight and highly customizable, improving user satisfaction and retention.
Top Android App Development Fundamentals You Must Use in 2025
Here is an in-depth review of our top 12 highly innovative fundamentals of Android mobile app development that just can not be missed in 2025:
1. Android Jetpack Compose
For creating native Android apps, Jetpack Compose is a cutting-edge UI toolkit. It uses a declarative approach to UI development, making it easier for developers to describe how the UI should look and behave.
This toolkit simplifies UI development by reducing boilerplate code and providing a more intuitive way to create interactive and dynamic interfaces.
2. Kotlin Programming Language
Kotlin is a statically typed programming language that is fully interoperable with Java, making it a preferred choice for Android development. It offers concise syntax, reducing the amount of code needed for common tasks.
Kotlin is designed to be null-safe, which helps in preventing null pointer exceptions, a common issue in Java. This enhances development speed and also reduces the likelihood of errors and the creation of robust applications.
3. Artificial Intelligence (AI) and Machine Learning (ML)
The rise of AI has resulted in Android apps increasingly incorporating AI and ML to offer features such as personalized recommendations, image recognition, and natural language processing.
TensorFlow and other ML frameworks have Android support, allowing developers to integrate machine learning models into their applications. As a result, apps now offer features such as predictive text, voice recognition, and recommendation systems, enhancing user engagement and satisfaction.
4. Android Instant Apps
Instant Apps allow users to experience an app without installing it. This is achieved through modularization, where specific features of the app can be accessed via deep links without requiring the user to download and install the entire app.
5. MotionLayout
MotionLayout is a part of the ConstraintLayout library and simplifies the creation of complex animations and transitions. Developers can define motion scenes to describe how UI elements move and transform between different states, providing smoother and more interactive user experiences.
6. Support for Foldable and Dual-Screen smartphones
As foldable and dual-screen Android smartphones become more common, developers are customizing their apps to take full use of these novel form factors.
Developers can create innovative and adaptable designs that seamlessly transition between different screen configurations, providing users with a more versatile and immersive experience.
7. Android App Bundles
App Bundles are a publishing format that includes all the resources and code necessary to deliver a personalized APK for each user’s device configuration. This helps reduce the size of app downloads and ensures that users receive optimized versions based on their device specifications.
8. Biometric Authentication
Android supports biometric authentication methods like fingerprint scanning and facial recognition for user authentication. Developers can integrate these features to enhance the security of their apps while providing a convenient and user-friendly authentication experience.
9. Android 15 Features
Android 15 includes a Theft Detection Lock that blocks unauthorized users plus Private Space which protects sensitive apps. Android 15 gives users control over video recording to protect privacy and lets them send satellite messages when there’s no cellular reception. Large screen users get better workflow through a taskbar always present at the bottom of their screen.
Developers now have more ways to work with fitness and health data through Health Connect and they receive modern Java 17 features from OpenJDK 17 integration. The system makes app document processing better within the platform and saves apps to free up device space.
These Android 15 updates create a safer operating system with superior speed and better tools for app builders.
10. Improved Security Measures
User data along with privacy benefit from the constant security upgrades which Android provides. Security development focuses on enhancing the app sandboxing functions as well as creating secure communication protocols and implementing encryption to protect user information integrity and confidentiality.
Recent Android version upgrades have fortified the security position of Android apps through new security features like runtime permissions and scoped storage along with privacy-oriented function additions.
11. 5G Technology Integration
With the rollout of 5G networks, developers are exploring ways to leverage faster data speeds for improved app performance. This includes optimizing content delivery, streaming high-quality media, and exploring new possibilities for real-time communication and data-intensive applications.
12. Location-Based Services
Apps use location data for various purposes, including personalized content delivery, location-aware notifications, and geotagging. Developers can integrate location-based services to enhance user engagement by delivering context-aware and relevant information based on the user’s location.
Top 7 Features and Resources to Consider When Choosing the Best Android App Development Framework
Here are the top resources and characteristics that you must consider before you choose the best Android app:
1. Integrated Development Environment
IDEs play a fundamental role in Android development. The complete set of tools integrated into these systems simplifies the development work and boosts productivity metrics.
Android developers have access to efficient code-producing tools and time-saving templates through IDEs which facilitate their programming process. The version control system integration deserves attention to boost development quality, development lifecycle efficiency, and developer productivity.
2. User Interface Design
Android Studio’s visual designer empowers professionals to develop Android apps using responsive and user-friendly UIs. It uses XML for layout design, which enhances developer productivity and satisfaction. You must consider criteria such as intuitive interfaces, accessibility features, documentation quality, and community support forums.
3. Programming Languages
Evaluation should focus on Java and Kotlin as the primary languages for Android app development. The sophistication of Kotlin features along with its developer productivity drives Google to approve Kotlin more frequently.
Find out how well Java features function within the software platform, especially regarding automated code completion and proper debugging abilities. These programming solutions additionally support C++ and JavaScript use for particular project needs.
4. Application Components
Android apps are developed using various components, such as activities, services, broadcast receivers, content providers, and fragments. Each component serves a distinct function within the app’s functionality. This is an important consideration, as it contributes to a well-organized architecture.
5. Application Programming Interfaces
Android has an extensive collection of APIs and libraries, which developers can access to make the most of device features like the camera, sensors, and location services. They can also integrate third-party libraries to speed up development and enhance app functionality.
6. Testing
Testing and debugging ensure the stability and functionality of Android apps during development. Developers utilize the best tools for testing on virtual devices and real devices for physical hardware testing. Unit, integration, and user interface testing are standard procedures in Android app development.
7. Integration with Version Control Systems
Integration with version control systems is important for managing code changes. The top Android app development frameworks should integrate with VCS providers and facilitate code collaboration through code review tools. It should also offer comprehensive documentation and community support for developers.
Conclusion
Android app development, whether for higher education, corporate training and development, or even business expansion, is rapidly expanding. You can now develop Android apps using innovative and technically advanced tools and resources that are emerging to enhance the process. You must consider relevant factors to save time and deliver high-quality, user-friendly apps.
Android’s success is mainly due to its open-source platform and affordable devices. By developing apps, you can capitalize on Android’s vast user base for quick market entry and eventually meet business objectives effectively.
If you are looking for professional assistance with Android app development services, reach out to the experts at Hurix Digital. They will help you with the latest tools that can deliver modern applications and ensure success in the market.
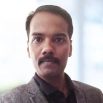
Currently serving as the Vice President of Technology Delivery Operations at HurixDigital, a prominent global provider of digital content and technology solutions for publishers, corporations, and educational institutions. With over 16 years of experience spanning EdTech and various domains, I hold certification as a SCRUM Product Owner (CSPO). My expertise includes operations, finance, and adept people management skills.